Wednesday, February 4, 2015
Android beginner tutorial Part 32 ListView widget
A ListView is a very useful and common widget that lets the user scroll through a list of items. There are multiple ways of using it. Today well see how to implement a ListView as a part of the layout of an Activity, how to display items from array in it and how to handle user item clicks.
Lets open our test application project and go to the acitivity_main.xml layout file.
Here add a simple LinearLayout, that contains a TextView and ListView. Give them ids and sizes as shown below:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/text"/>
<ListView
android:id="@+id/list"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
</LinearLayout>
Now go to MainActivity.java class.
Declare an array of contact names:
final String[] contacts = {"Fernando Reigle","Lorrie Chamberland","Earlene Cully","Lorrie Down",
"Serena Adolph","Sofia Tilford","Tyrone Heiner","Mathew Betterton","Fernando Padula","Hugh Gassaway",
"Jamie Tiffin","Nelson Velazco","Liza Goudy","Christian Donahoo","Ted Baisley","Jessie Holte","Christian Corrao",
"Saundra Swoboda","Margery Canez","Lance Midyett","Jamie Burlew","Allyson Trudel","Tyrone Spofford"};
Declare a TextView variable:
public TextView text;
In the onCreate() function we set the value of "text" to reference the "text" TextView in the layout. We declare a "list" variable which references the "list" ListView in the layout, then set an adapter for it using a setAdapter() method, and add a click listener using setOnItemClickListener():
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
text = (TextView) findViewById(R.id.text);
ListView list = (ListView) findViewById(R.id.list);
list.setAdapter(new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, contacts));
list.setOnItemClickListener(onClick);
}
The OnItemClickListener instance contains a public function onItemClick(), which updates the text of our TextView. It displays the name of the selected contact using the provided "position" value, which is the index value of that contact in the array:
public OnItemClickListener onClick = new OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
text.setText("Selected: " + contacts[position]);
}
};
Full code:
package com.kircode.codeforfood_test;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.TextView;
public class MainActivity extends Activity{
final String[] contacts = {"Fernando Reigle","Lorrie Chamberland","Earlene Cully","Lorrie Down",
"Serena Adolph","Sofia Tilford","Tyrone Heiner","Mathew Betterton","Fernando Padula","Hugh Gassaway",
"Jamie Tiffin","Nelson Velazco","Liza Goudy","Christian Donahoo","Ted Baisley","Jessie Holte","Christian Corrao",
"Saundra Swoboda","Margery Canez","Lance Midyett","Jamie Burlew","Allyson Trudel","Tyrone Spofford"};
public TextView text;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
text = (TextView) findViewById(R.id.text);
ListView list = (ListView) findViewById(R.id.list);
list.setAdapter(new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, contacts));
list.setOnItemClickListener(onClick);
}
public OnItemClickListener onClick = new OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
text.setText("Selected: " + contacts[position]);
}
};
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
The results look like this:
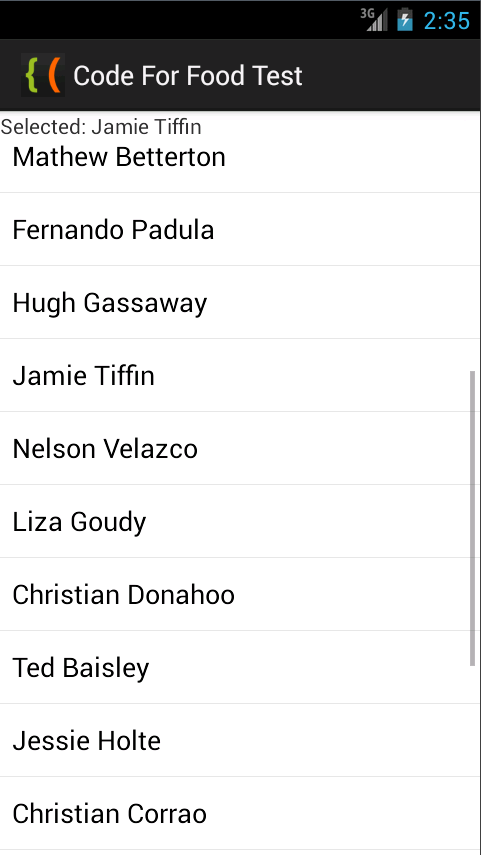
Thanks for reading!